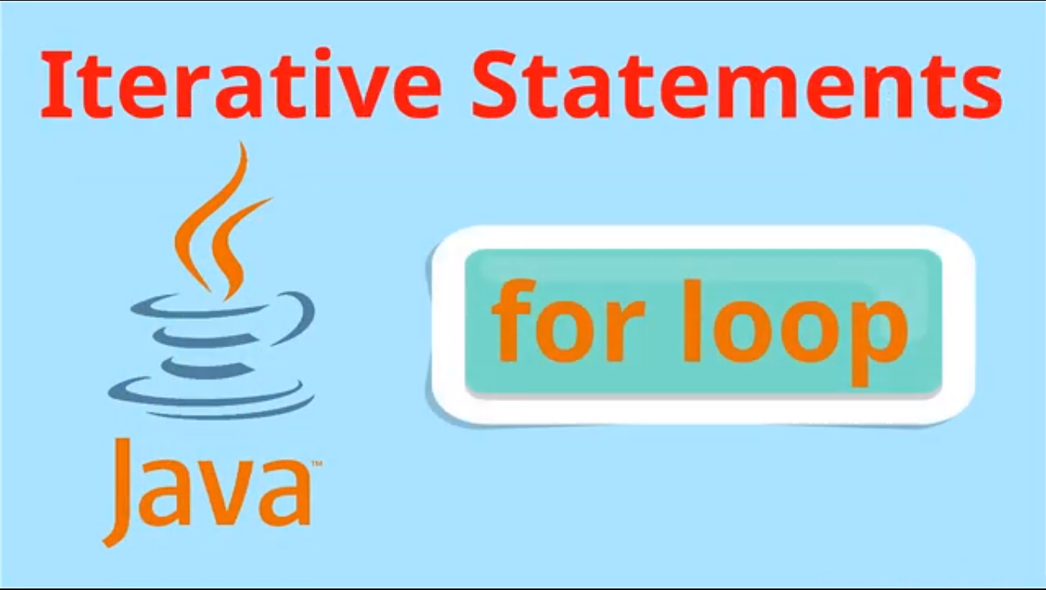
Loops in Java
- Categories Iterative Statements, For & While, Java
In this post we will cover loops in java. You can watch our videos on Java For Loop – Click Here
Q.1 What is iteration? Give any 2 iteration statements in Java?
Ans. Iteration means looping. When we need to execute a block of code several number of times, it is often referred to as a iteration or loop. Java has very flexible three looping mechanisms. You can use one of the following three loops:
- while Loop
- do..while Loop
- for Loop
Q.2 What is for loop in Java?
Ans. The for loop is a looping construct which can execute a set of instructions a specified number of times.
It’s a counter controlled loop, with 3 parts
- Starting initialization, which executes once before the loop begins. The section can also be a comma-separated list of expression statements.
- Test expression. As long as the expression is true, the loop will continue. If this expression is evaluated as false the first time, the loop will never be executed.
- Incr/decr expression automatically executes after each repetition of the loop body.
- Syntax
for (starting initialization ; test expression ; <incr/decr expression>)
{
}
Q.3 State the difference between entry controlled loop and exit controlled loop.
Ans.
entry controlled loop | exit controlled loop |
---|---|
1. The loop condition is checked before executing the body of the loop | 1. The loop condition is checked after executing the body of the loop. |
2. Example: While loop and for loop | 2. Example: do-while loop |
In an entry controlled loop, the loop condition is checked before executing the body of the loop. While loop and for loop are the entry controlled loops in Java. In exit controlled loops, the loop condition is checked after executing the body of the loop. do-while loop is the exit controlled loop in Java
Q.4 What is an infinite loop?
Ans. The loop which never ends and does not reach to the test condition is known as infinity loop. It is also known as unending loop.
Example: for(int y=1; y<=10; y–) ;
This loop ‘y’ starts from ‘1’ and goes upto 10 but the update statement ‘y- -‘ makes the loop infinite or unending because the condition y<=10 will always be true.
Q.5 What is the difference between while and do while loop?
Ans.WHILE DO-WHILE Condition is checked first then statement(s) is executed. Statement(s) is executed atleast once, thereafter condition is checked. It might occur statement(s) is executed zero times, If condition is false. At least once the statement(s) is executed. No semicolon at the end of while.
while(condition)Semicolon at the end of while.
while(condition);If there is a single statement, brackets are not required. Brackets are always required. Syntax
while (expression)
{
statement(s)
}do
{
statement(s)
} while (expression);
Q.6 What happens if we put a ; at the end of for loop?
Ans. If a semicolon is placed after the for() statement then semicolon is treated as the body of the loop and the for statement executes the null loop. Once the null loop is finished, it will execute the statement(s) following the for() statement.
Example:
for(i=0; i<3; i++);
{
System.out.println(“i= ” +i);
}
Output: i=3
Q.7 What is difference between break and continue statement?
Ans.Break Continue Break keyword is used to break or stop a loop execution Continue terminates the current iteration and passes the control to the next iteration of the loop Control passes to next statement following the loop Control passes to next iteration of the loop. Break can be used with switch statement and loops Continue is used along with loops only e.g.
for ( int x = 0;x < 10;x++) {
if(x < 5)
System.out.println (“Less than 5”);
else
break;
}e.g.
for(int x = 0;x < 10;x++) {
if(x%2 == 0)
print (“Even”);
else
continue;
}
You may also like

Questions on Encapsulation

Questions on Library classes
