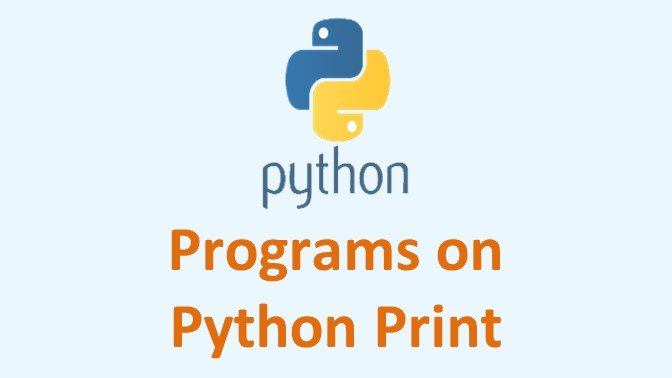
CBSE Python Questions on Print.
- Categories Python, Python Basics, Python
CBSE Python Questions on Print.
1. Find and write the output of the following Python code:
Number = [9,18,27,36] for N in Number: print (N,"#",end = " ") print ()
Ans.
9 # 18 # 27 # 36 #
2. Find and write the output of the following Python code:
print("Simply coding Python") a = 5 print("a =", a) b = a print('a =', a, '= b')
Ans.
Simply coding Python a = 5 a = 5 = b
3. Find and write the output of the following Python code :
Values = [10,20,30,40] for val in Values: for I in range (1,val%9): print (I,"*", end= " ") print ()
Ans.
1 * 1 * 2 * 1 * 2 * 3 *
4. Find and write the output of the following Python code :
a=4 a+=a*a print(a)
Ans. 20
5. Find and write the output of the following Python code :
for i in range(4): print (i) for i in range(5,8): print (i*5)
Ans.
0 1 2 3 25 30 35
6. Find and write the output of the following Python code :
list1 = [84, 42, 63, 34, 55] list2 = list1 list2[3] =list2[2]//2; print ("list1= ", list1)
Ans.
list1= [84, 42, 63, 31, 55]
7. Find and write the output of the following Python code :
nameList = ['Priti', 'Sharda', 'Bob', 'Kabir'] pos = nameList.index("Bob") print (pos * 5 )
Ans. 10
8. Find and write the output of the following Python code :
list1 = [2016, 2022, 2010, 2020] list2 = ['Priti', 'Sharda', 'Kabir'] print ("list1 + list2 = : ", list1 + list2) print ("list1 * 2 = : ", list1 * 2)
Ans.
[2016, 2022, 2010, 2020, ‘Priti’, ‘Sharda’, ‘Kabir’]
[2016, 2022, 2010, 2020, 2016, 2022, 2010, 2020]
9. Find and write the output of the following Python code :
list1 = [45, 8, 73, None, (31, 72, 53, 41, 25)] list2= (65,52,47) print (list1,list2)
Ans.
[45, 8, 73, None, (31, 72, 53, 41, 25)] (65, 52, 47)
10. Find and write the output of the following Python code :
a=3 b='1' c=a-2 d=a-c e='study' f="Sharda" g='python' print(a,b,c,d,",",f,e,g)
Ans.
3 1 1 2 , Sharda study python
You may also like

Python Data Types
15 June, 2021

Python Tokens
15 June, 2021

Practice short problems on Python Random Module
25 April, 2020