
Java Operators and Operator precedence questions
- Categories Java, Operators, Java Operators
Today we are going to practice questions on Java Operators and its precedence. Before you attempt the questions, do a quick review of these two topics by going through our video link here.
- Name the type of operators listed below (assignment, relational, logical etc):
- <
- ++
- &&
- ?:
Ans.
- Relational Operator
- Arithmetic Operator (Increment, unary)
- Logical operator (and)
- Ternary operator
- Name the type of operators listed below (assignment, relational, logical etc):
- !
- ==
- %
- =
Ans.
- Logical Operator
- Relational Operator
- Arithmetic Operator (Modulus)
- Assignment operator
- Operators with higher precedence are evaluated before operators with relatively lower precedence. Arrange the operators given below in order of higher precedence to lower precedence.
(i) && (ii) % (iii) >= (iv) ++
Ans.
(i) ++ (ii) % (iii) >= (iv) &&
- Arrange the operators given below in order of higher precedence to lower precedence.
(i) () (ii) ?: (iii) == (iv) | |
Ans.
(i) () (ii) == (iii) | | (iv) ?:
- Arrange the operators given below in order of higher precedence to lower precedence.
(i) + (ii) && (iii) != (iv) ++
Ans.
(i) ++ (ii) + (iii) != (iv) &&
- What will be the output of the following. if x=5 initially?
(i) 5 * ++x
(ii) 5 * x++
Ans:
(i) 30
(ii) 25
- What is the result stored in x, after evaluating the following expression?
int x = 5; x = x++ * 2 + 3 * –x;
Ans.
x=-8
- Give the output of this code
int a = 9; a++; System.out.println (a); a -= a-- - --a; System .out.println (a);
Ans:
10
8
Explanation
a = 9; a++; // a = 10 SOP (a) // Prints 10 a -= a-- - --a; a = 10 – (10 – 8) a = 10 -2 = 8 SOP (a) // Prints 8
- Evaluate the following expression: when a=10, b=8
i) ++a-b– ii) a%b++
iii) a*=b+5 iv) x=69>>>2
Ans
(i) 3 (ii) 2 (iii) 130 (iv) 17
- If m=5 and n=2 output the values of m and n after execution in (i) and (ii).
(i) m -= n;
(ii) n = m + m/n;
Ans:
(i) the value of m is 3 and n is 2
(ii) The value of m is 5 and n is 7
- What will be the output of the following code?
int k = 5, j = 9; k += k++ – ++j + k; System.out.println(“k=” +k); System.out.println(“j=” +j);
Ans.
k= 6
j= 10
- What is the value of y after evaluating the expression given below
y+=++y + y– + –y; when int y=8.
Ans.
y=33
- Give the output of the following expression:
a+=a++ + ++a + –a + a–; when a=7.
Ans.
a=39
- If int y=10 then find y and z after executing this statement:
int z=(++y * (y++ +5));
Ans.
y=12 z=176
- What will be the result stored in x after evaluating the following expression?
int x=4; x += (x++) + (++x) + x;
Ans.
x=20
- What is the value of y after evaluating the expression given below?
y += ++y + y– + –y; when int y = 8.
Ans:
y = 8 + 9 + 9 + 7
y = 33
- What is the value of x1 if x=5 ?
x1=++x – x++ + –x
Ans.
x1= ++x – x++ + –x
x1= 6 (x is incremented to 6) – 6 (x is incremented to 7) + 6 (x is decremented to 6)
= 6
- Give the output of the following expression :
a+=a++ + ++a + -–a + a-– ; when a = 7
Ans.
a+=a++ + ++a + –-a + a–- ; a = 7 + (a++ + ++a + -–a + a–-); a = 7 + (7 + 9 + 8 + 8); a = 39
- If int y = 10 then find int z = (++y * (y++ + 5));
Ans.
Increment operator has the highest precedence. So, ++y and y++ will be evaluated starting from left.
In ++y, the value of y will be incremented to 11 and then 11 will be used in the expression.
When y++ is evaluated, the current value of y i.e. 11 will be used and then y will be incremented from 11 to 12.
int z = (++y * (y++ + 5)); = 11 * (11 + 5 ) = 11 * 16 = 176
You may also like

Java Operators
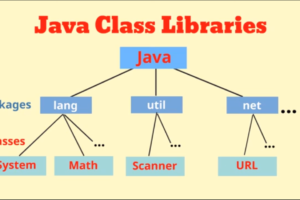
Java Class Libraries and Packages
