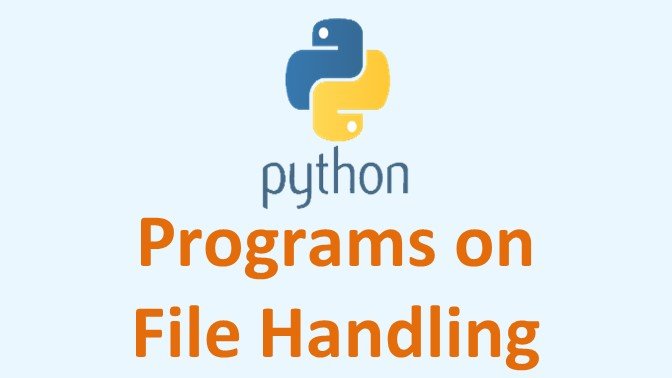
Programs on Python File Handling
- Categories Python, Python File Handling
Programs on Python File Handling:
1.Write a program to display all the records in a file “python.txt” along with line/record number?
Ans.
fh=open("python.txt","r") count=0 rec="" while True: rec=fh.readline() if rec=="": break count=count+1 print(count,rec) fh.close()
2.A text file “PYTHON.TXT” contains alphanumeric text. Write a program that reads this text file and prints only the numbers or digits in the file.
Ans.
fh=open(“python.txt","r") rec=fh.read(); for a in rec: if a.isdigit(): print(a,end=' ') fh.close()
3.Write a function LongLine() that accepts a filename and prints the file’s longest line with its length?
Ans.
def get_longest_line(filename): mydict = {} for line in open(filename, 'r'): mydict[len(line)] = line return mydict[sorted(mydict)[-1]] get_longest_line('car.txt')
4.Write a program to count the number of upper-case alphabets present in a text file “PYTHON.TXT”
Ans.
def uppercount(): upper=0 f1=open("PYTHON.txt",'r') line=f1.read() for i in line: if (i.isupper()): upper+=1 print("Total no. of upper-case alphabets :",upper) uppercount()
5.Write a program to count the words “to” and “the” present in a text file “python.txt”.
Ans.
fname = "python.txt" num_words = 0 with open(fname, 'r') as f: for line in f: words = line.split() num_words += len(words) print("Number of words:") print(num_words)
6.Given a text file car.txt following information of car ‘carNo,carname,containing mileage’. Write a python function to display details of all those cars whose mileage is fron 100 to 150.
Ans.
def display(): file=open("car.txt",'r') lines=file.readlines() file.close() for line in lines: x=line.split(',') mileage=(int) (x[2]) if mileage>100 and mileage<=150: print(line) display()
7.Write a program to print following type of statistics for the given file.
A text file ‘car.txt’ contain following information of car carNo,carname,containing mileage. Write a python function to display :
- Number of lines in the file
- Number of empty lines
- Average characters per non-empty line
Ans.
fp1=open("car.txt","r") lines=fp1.readlines() totallines=len(lines) blank=0 char=0 for i in lines: char+=len(i) i = i.strip() if len(i)==0: blank+=1 fp1.close() print (totallines,"lines in the file") print (blank,"Empty lines") print (char/(totallines-blank),"average characters per non-empty line")
You may also like
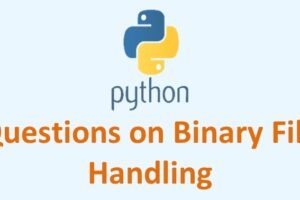
Questions on Binary File Handling in Python
13 June, 2021

Questions on Text File Handling in Python
13 June, 2021
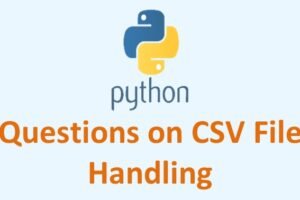
Questions on CSV File Handling in Python
12 June, 2021