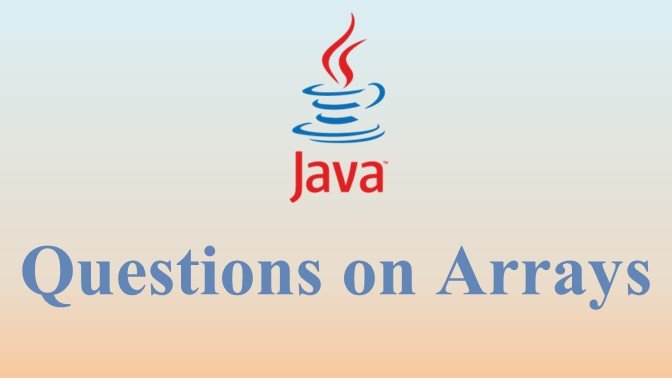
Arrays in Java
- Categories Arrays in Java, Arrays, Arrays, Java
In this post we will cover Arrays in java. You can watch our videos on Array in Java with Practice Short Questions And 2D Arrays in Java
Q. 1 What are arrays?
Ans. An array is defined as set of homogenous data elements (same name and same data type) stored in a contiguous memory location.
- All elements share same data type e.g. array of int
- Entire collection identified with single name
- In Java, an array is a reference data type
- Array size is finite
- Arrays can be single dimensional, double dimensional or multi-dimensional too.
Example : int ar[ ]= {2, 4, 6, 7, 8};
In this example element 2, 4, 6, 7, 8 are stored under the array ‘ar’ in different memory indexes.
Q. 2 How do we declare an array?
Ans. We can declare an array separately or do declaration + initialization together.
char [] upperA; // Only Declaration
upperA = new char[26]; // Only Initialization
int marks[];
marks = new int[10];
int[] numbers = new int[10]; // Declaration + Initialization
You can also initialize an array with set values.
Example:
int[] numbers = {10,30,100};
char upperA[]; = {‘A’,’E’,’O’,’I’,’U’};
Q. 3 Why do we use an array? Explain using one example.
Ans. Arrays are very useful to store large amount of elements of same data type under one variable
Example : int ar[ ]= {2, 4, 6, 7, 8};
In this example elements 2, 4, 6, 7, 8 are stored under the array ‘ar’ in different memory indexes.
Q. 4 How can we get number of elements in the array?
Ans. An array has a public constant length for the size of an array.
Example: Int X = student.length;
- Array is a reference data type, not an object. Therefore, we do not use a method call.
- This approach is particularly useful when the size of an array may change, or is not known in advance.
- Array Index or Subscript is between : 0 – (array.length – 1)
Q. 5 What is an array Index?
Ans. Index of an array is the location of a value in a particular array
- Individual elements are accessible via index or subscript indicating the element’s position within the collection
- Index of an array start with 0
Example:Index 0 1 2 3 4 5 6 7 8 Array Elements 23 4 15 34 6 10 11 25 4
Q. 6 What happens if you try to access element at index arr.length or beyond?
Ans. If we try to access any element at arr.length or beyond we get an exception – ArrayIndexOutOfBoundsException.
Q. 7 What is a double Dimensional Array? Write the syntax for same.
Ans. The array which creates memory locations/indexes Row wise and Column wise (i.e. in two dimensions) is known as double dimensional array or multi-dimensional array.
Syntax:
data type array_name/variable[ ][ ] = new data type[number of Rows][number of Columns];
Or
data type [ ][ ] array_name/variable = new data type[number of Rows][number of Columns];
Example:
int x[ ][ ]= new int[3][3]; or
int [ ]x= new int[3][3]; or
int x[ ][ ];
x= new int[3][3];
Q. 8 What is sorting? List the type of sorting.
Ans. The method of arranging elements of an array in ascending order or in descending order is known as sorting.
Types of sorting:
- Selection
- Bubble
- Insertion
- Merge
- Quick
- Shell
Q. 9 What is bubble sort?
Ans.
- In this method two adjacent elements of the array are compared each time and
- If they are not at their proper places (or indexes)
- The elements are interchanged (or swapped) to place them at the actual indexes
- This process goes for all the elements of the array and finally the array will be in sorted form. This is known as bubble sort technique
Q. 10 What is searching?
Ans. The process of finding an element from either an ordered or unordered list of data or array is known as searching.
The searching method is divided in following two categories:
- Linear search
- Binary search
Q. 11 Differentiate between searching and sorting?
Ans. In searching, we are provided with an array which contains multiple elements and asked to find the index of a specific element.
In sorting, an array is provided and we are asked to order the elements in either ascending or descending order.
Q. 12 What do you mean by Binary Search?
Ans: Binary Search
- This search technique searches the given ITEM in minimum possible time.
- The Binary search requires that the array must be sorted.
- The search ITEM is compared with middle element of the array.
- If the ITEM is more then the middle element later part of the arrays becomes the new array segment.
- The same process is repeated until either the ITEM is found or the array segment is reduce to single element.
Q. 13 Differentiate between linear search and binary search techniques?
Ans. In linear search each elements of the array is compared with the given item to be searched for one by one while binary search searches for the given item in a sorted array. The search segment reduces to half at every successive stage.
Q. 14 Name the search or sort algorithm that:
- Makes several passes through the array, selecting the next smallest item in the array each time and placing it where it belongs in the array.
- At each stage, compares the sought key value with the key value of the middle element of the array.
Ans.
- Selection Sort
- Binary Search
Q. 15 Write one difference between Linear Search and Binary Search?
Ans. Linear search can be used with both sorted and unsorted arrays. Binary search can be used only with sorted arrays.
You may also like

Questions on Encapsulation

Questions on Library classes
