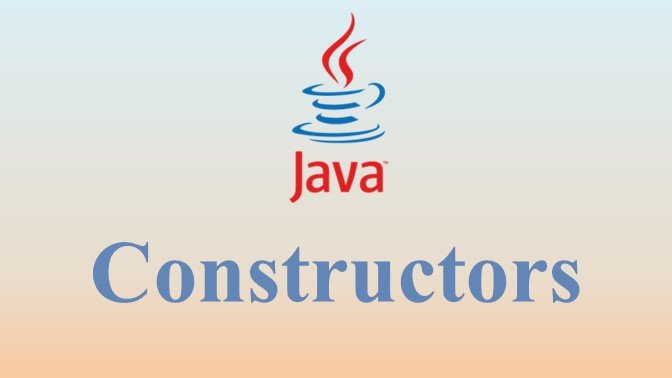
Constructors
- Categories Constructors, OOPS, Java
In this post we will cover Constructors in java. You can watch our videos on Revise Java Constructors in 5 minutes – Click Here
Q. 1 What is constructer? When is it invoked? Or Write two characteristics of a constructor. Give example.
Ans.
- Constructor is a special method(or function) defined with the same name as of the class.
- It has no return type, not even void.
- It is automatically invoked wherever an object of that class is created.
- // Example: A simple constructor.
class MyClass {
int x;
// Following is the constructor
MyClass() {
x = 0;
}
}
Q. 2 What is the difference between Constructor and method/function?
Ans.
Constructor | Method/function |
---|---|
1. It is always defined by the same name as of the class | 1. It is defined by any name except class name and keyword. |
2. It has no return type not even void data type | 2. It must begin with either void or a valid return data type (such as int, float, char etc.). |
3. It initializes the data members of the class. | 3. A method contains one or more than one valid Java statements as its body. |
4. It can not be invoked/called like a method because a constructor automatically runs whenever an object of the class is created. | 4. A method must be invoked or called either by using object of the class or directly. |
Q. 3 What are different types of constructors?
Ans. There are 3 different types of constructors
- Default Constructor
- Parameterized Constructor
- Copy Constructor
Q. 4 What is Java default constructor?
Ans. There are 2 types of Default constructor one provided by Java itself and one implemented by the programmer.
- If a class does not have any constructor, the Java compiler will automatically provide one non-parameterized-constructor (or default-constructor) for the class in the byte code.
- The default constructor initializes the member variables to 0.
- The access modifier of the default constructor is the same as the class itself.
- A programmer can give his own implementation of default constructor and in that case it overrides they system provided constructor.
Q. 5 What is the difference between parameterized and copy constructor?
Ans.
- Parameterized constructor: When a constructor takes in parameters within the parenthesis it is known as parameterized constructor.
- Copy constructor: Copy Constructor is a special type of constructor that takes same class as argument. Copy constructor is used to provide a copy of the specified object.
- Example:
public class simply { int a; char c; simply( ){ // Default Constructor a= 10; } simply(char n){ // Parameterized Constructor c=n; } simply(simply s) // Copy Constructor { int a= s.a; } }
Q. 6 Explain the concept of constructor overloading with an example.
Ans. Presence of two or more constructors in a class is known as constructor overloading.
Example:
class stud
{
static int year;
int Roll_No;
stud( ){ // constructor 1. This is default or non-parameterized constructor
year= 2019;
}
stud( int n){ // constructor 2. This is a parameterized constructor
Roll-No= n;
}
}
Q. 7 What is this keyword in Java?
Ans. this keyword in Java is a special keyword which can be used to represent current object or instance of any class in Java.It stores the address of current calling object. It is also known as reference or pointer to current object.
Any instance method can be called as this.method() and any instance variable var as this.var.
public class Actor
{
string lastName;
public Actor(String lastName)
{
this.lastName = lastName;
}
}
Q. 8 What is parameterized constructor with example?
Ans. When a constructor takes in parameters within the parenthesis it is known as parameterized constructor.
Example:
public class simply
{
int roll_no;
char grade;
simply( ){ // Default Constructor
roll_no= 10;
}
simply(char n){ // Parameterized Constructor
grade =n;
}
}
Q. 9 What are temporary instance of a class and how it is created? Give one example also.
Ans.
- It is an anonymous instance that is created to perform only one particular job within a single statement.
- Temporary instances are created by calling or invoking constructor of a class using explicit method within the statement which performs some specific job.
- Example: new Obj().Show();
Q. 10 Fill in the blanks
- A member function having the same name as that of the class name is called ____.
- A constructor has ____ return type.
- A constructor is used when ______ is created
- A constructor without any argument is known as ___________
- ____________ constructor creates objects by passing value to it.
- The ______ keyword refers to the current object.
- The argument of a copy constructor is always passed by __________.
- The constructor generated by the compiler is known as ___________.
Ans.
- A member function having the same name as that of the class name is called constructor.
- A constructor has return no
- A constructor is used when an object is created
- A constructor without any argument is known as non-parameterised constructor.
- Parameterised constructor creates objects by passing value to it.
- The this keyword refers to the current object.
- The argument of a copy constructor is always passed by reference.
- The constructor generated by the compiler is known as default constructor.
Q. 11 State True or False
- The compiler supplies a special constructor in a class that does not have any constructor.
- A constructor is not defined with any return type.
- Every class must have all types of constructors.
- A constructor is a member function of a class.
- Constructor is used to initialize the data members of a class.
- A constructor may have different name than the class name.
- A constructor is likely to be defined after the class declaration.
- Copy constructor copies functions from one object to another.
Ans.
- True
- True
- False
- True
- True
- False
- False
- False
You may also like

Questions on Encapsulation

Questions on Library classes
