
Python Programs on Recursion
- Categories Python, Python Recursion
Python Programs on Recursion:
1.Write a program using a recursive function to print Fibonacci series up to nth term.
Ans.
def fibo(n): if n==1: return 0 elif n == 2: return 1 else: return fibo(n-1)+fibo(n-2) n=int(input("enter no of fibonnaci nos to be printed:")) for i in range(1,n+1): print(fibo(i),end=',')
2.Write a recursive function to implement binary search algorithm.
Ans.
def binsearch(array,key,low,high): if low>high: return -1 mid=int((low+high)/2) if key==array[mid]: return mid elif key <array[mid]: high = mid-1 return binsearch(array,key,low,high) else: low=mid+1 return binsearch(array,key,low,high) sortedarray=[10,5,12,15,20,31,34] item= int(input("enter search item:")) res=binsearch(sortedarray,item,0,len(sortedarray)-1) if res>=0: print("Found") else : print ("Not Found")
3.Write recursive function to take in a string to print it backwards.
Ans.
def backprint(S): n = len(S) if n == 0: return print(S[-1], end = '') backprint (S[0:-1]) # _main_ s = input("Enter a string : ") backprint(s)
4.Write a program to show the use of recursion in calculation of power of a number.
Ans.
def power(a, b) : if b == 0 : return 1 else : return a * power(a, b-1) n = int (input("Enter number :")) p = int(input ("Enter power of : ")) result = power(n,p) print(n, "raised to the power of", p, "is", result)
5.Write recursive code to compute greatest common divisor of two numbers.
Ans.
def gcd(a,b): if(b==0): return a else: return gcd(b,a%b) n1=int(input("enter first number:")) n2=int(input("enter second number:")) d=gcd(n1,n2) print("GCD of", n1, "and",n2,"is:",d)
6.Write recursive code to compute and print sum of squares of numbers. Value of n is passed as parameter.
Ans.
def sqsum(n): if n==1: return 1 return n*n+sqsum(n-1) n=int(input("enter value of n:")) print(sqsum(n))n
You may also like

Solve any character pattern program in python
3 June, 2021
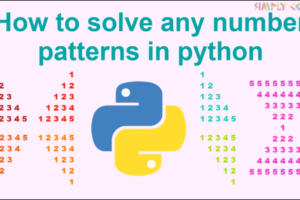
Solve any number patterns programs in python
3 June, 2021

Star Pattern Programs In Python
20 May, 2021