
Python Programs on Tuples
- Categories Python, Python Tuple
Python Programs on Tuples:
1. Write a Python program to test if a variable is a list or tuple or a set.
Ans.
#x = ['a', 'b', 'c', 'd'] #x = {'a', 'b', 'c', 'd'} x = ('tuple', False, 3.2, 1) if type(x) is list: print('x is a list') elif type(x) is set: print('x is a set') elif type(x) is tuple: print('x is a tuple') else: print('Neither a list or a set or a tuple.')
2. Write a Python program to sort a list of tuples by the second Item.
Ans.
def Sort_Tuple(tup): lst = len(tup) for i in range(0, lst): for j in range(0, lst-i-1): if (tup[j][1] > tup[j + 1][1]): temp = tup[j] tup[j]= tup[j + 1] tup[j + 1]= temp return tup tup =[('for', 2), ('is', 9), ('to', 7),('Go', 5), ('or', 15), ('a', 13)] print(Sort_Tuple(tup))
3. Write a Python program which accepts a sequence of comma-separated numbers from user and generate a list and a tuple with those numbers.
Ans.
values = input("Input some comma seprated numbers : ") list = values.split(",") tuple = tuple(list) print('List : ',list) print('Tuple : ',tuple)
4. Write a python program to print sum of tuple elements.
Ans.
test_tup = (7, 5, 9, 1, 10, 3) print("The original tuple is : " + str(test_tup)) res = sum(list(test_tup)) # printing result print("The sum of all tuple elements are : " + str(res))
5. Write a Python program to sort a list of tuples alphabetically
Ans.
def SortTuple(tup): n = len(tup) for i in range(n): for j in range(n-i-1): if tup[j][0] > tup[j + 1][0]: tup[j], tup[j + 1] = tup[j + 1], tup[j] return tup tup = [("Amaruta", 20), ("Zoe", 32), ("Akshay", 25), ("Nilesh", 21), ("C", "D"), ("Penny", 22)] print(SortTuple(tup))
6. Write a python program to Check if the given element is present in tuple or not.
Ans.
test_tup = (10, 4, 5, 6, 8) # printing original tuple print("The original tuple : " + str(test_tup)) N = int(input("value to be checked:")) res = False for ele in test_tup : if N == ele : res = True break print("Does contain required value ? : " + str(res))
You may also like

Python Tuples
15 June, 2021

Solve any character pattern program in python
3 June, 2021
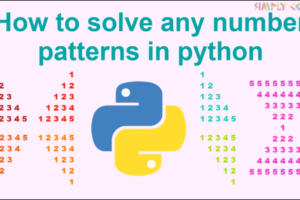
Solve any number patterns programs in python
3 June, 2021