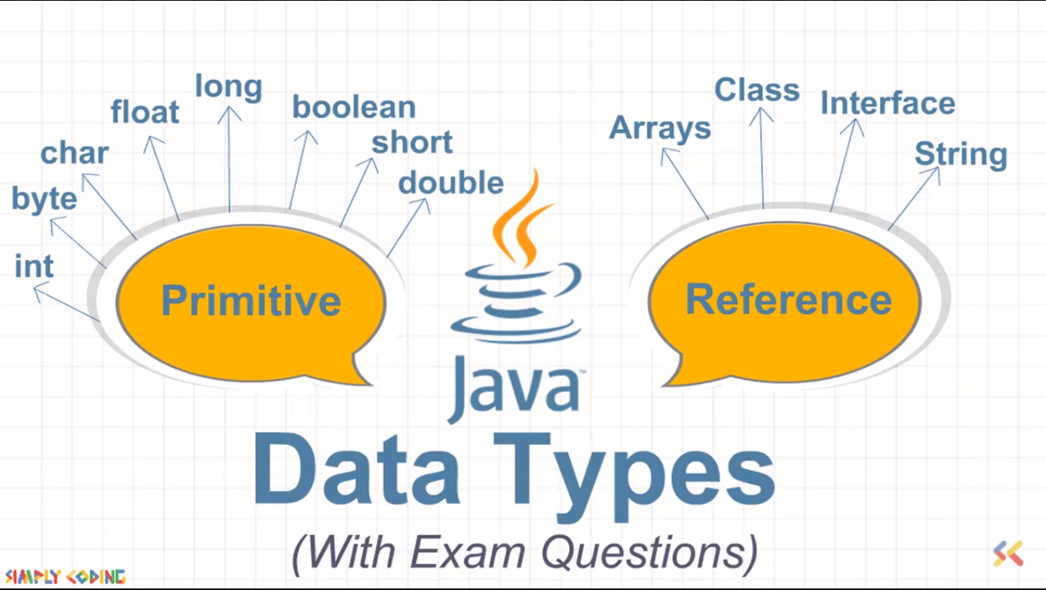
Java Data Types
- Categories Uncategorized
Here we are going to learn above Java Data Types. You can watch our video on Java Data Types- Click Here
Q. What is data type?
Ans. Data type refers to the type of data that can be stored in a variable. Java has 2 data types One is primitive and other is reference data type.
Q. What is Primitive Data Type?
Ans. Primitive Data Types are Data types which are built into Java language. E.g. int,char etc. Java has following data type

Q. What are ASCII data type and Unicode datatype?
Ans.
- only 1 byte was used to store character data.
- ASCII or (American Standard Code for Information Interchange) character encoding scheme was used to representing English characters as
numbers, with each letter assigned a number from 0 to 127. - For e.g.

- However it had its limitation as 1 byte could only encode 255 characters so all other languages were not represented.
- Then Unicode was introduced as an international encoding standard
- it uses 2 bytes and could encode different languages and scripts, by which each letter, digit, or symbol is assigned a unique numeric value that applies across different platforms and programs.
Q. What is reference data type? Give 2 examples of reference data types?
Ans. Reference Data Types are user created datatype using the primitive data types. Reference data type stores address or reference to data.
Example: Class, Interface and Array.
Q. Why java is call as strongly typed language?
Ans. variables can ONLY take value that matches the variable’s data type.
The compiler ensures that the program does not try to assign data of the wrong type to the variable.
For e.g. in statement below, you cannot assign double to an int variable. It will give an error.
int val1 = 12.6; // Error
Q. What is Type casting? What are 2 types of casting available in Java?
Ans. To convert one predefined data type to another data type is known as Type Conversion or Type Casting.
There are two type of type casting:
- Explicit Type Casting
- Implicit Type Casting
Q. What is Explicit and Implicit Type casting?
Ans.
Implicit Typecasting :
- When a data type of lower size (occupying less memory) is assigned to a data type of higher size, it is done implicitly by the JVM.
- The lower size is widened to higher size.
- This is called as Implicit Typecasting and is also named as automatic type conversion.
- Implicit type casting occurs if you assign any of these data types to higher data types
byte –> short –> int –> long –> float –> double - e.g
int x = 10; // occupies 4 bytes
double y = x; // occupies 8 bytes
System.out.println(y); // prints 10. - Note A boolean value cannot be assigned to any other data type.
Explicit Typecasting :
- A data type of higher size (occupying more memory) cannot be assigned to a data type of lower size.
- This is not done implicitly by the JVM and requires explicit casting; a casting operation to be performed by the programmer.
- The higher size is narrowed to lower size.
- A narrowing primitive conversion may lose information about the overall magnitude of a numeric value and may also lose precision and range.
- e.g.
double x = 10.5; // 8 bytes
int y = x; // 4 bytes ; raises compilation error
int y = (int)x; // ok. Explicit Typecasting
You may also like

XML Prolog

XML Elements, Tags and Attributes
